气泡对话框是覆盖在当前界面之上的弹出框,可以相对组件或者屏幕显示。显示时会获取焦点,中断用户操作,被覆盖的其他组件无法交互。气泡对话框内容一般简单明了,并提示用户一些需要确认的信息。
接口说明
构造方法
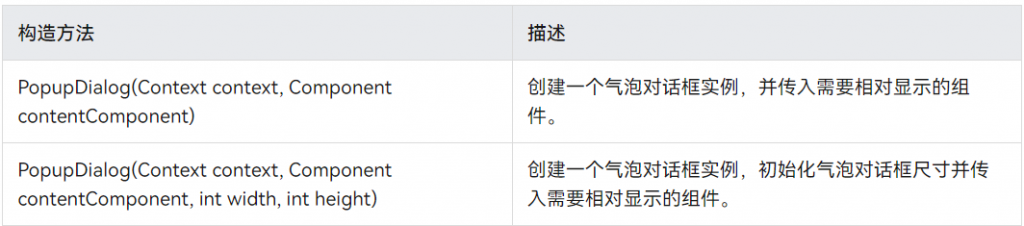
常用方法
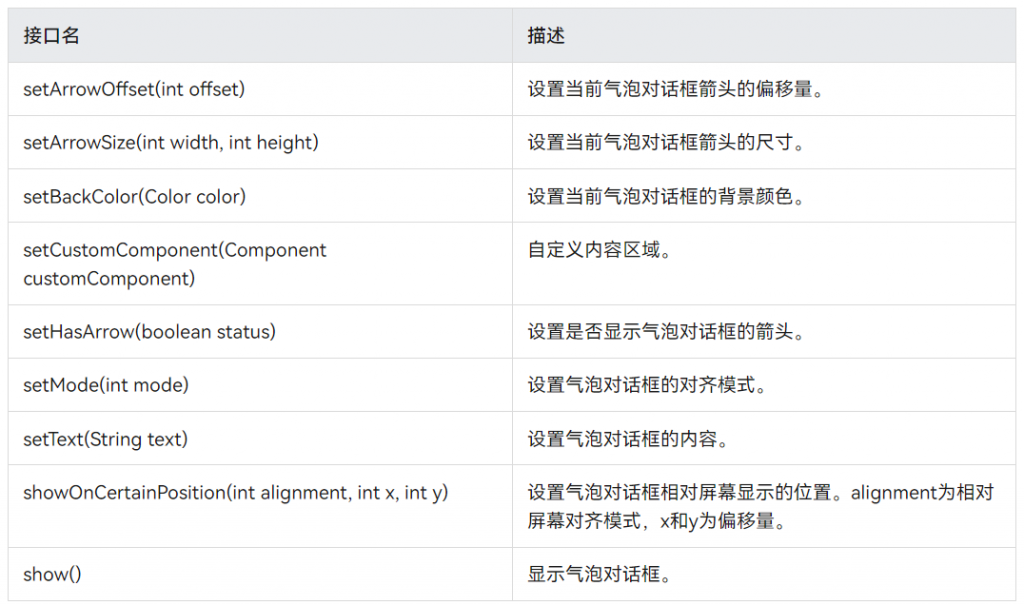
创建和使用
创建参照的组件
在创建之前我们需要先获取一个用来参照的组件,因为气泡对话框的主要特点就是相对其他组件进行显示的。XML代码如下:
<?xml version="1.0" encoding="utf-8"?>
<DependentLayout
xmlns:ohos="http://schemas.huawei.com/res/ohos"
ohos:height="match_parent"
ohos:width="match_parent"
ohos:background_element="#3C3F41">
<Button
ohos:id="$+id:target_component"
ohos:height="match_content"
ohos:width="match_content"
ohos:text="Click Here"
ohos:text_color="white"
ohos:text_size="18fp"
ohos:background_element="#1E90FF"
ohos:top_padding="8vp"
ohos:bottom_padding="8vp"
ohos:left_padding="16vp"
ohos:right_padding="16vp"
ohos:top_margin="200vp"
ohos:horizontal_center="true"/>
</DependentLayout>
创建气泡对话框
PopupDialog popupDialog = new PopupDialog(getContext(), component);
该构造方法中的第二个参数为Component对象,是气泡对话框显示时参照的组件,气泡对话框会根据对齐模式在这个参照组件的相对位置进行显示。
PopupDialog popupDialog = new PopupDialog(getContext(), component, 100, 100);
调用这个构造方法,是在上文两个参数的构造方法的基础上设置气泡对话框的尺寸。
使用气泡对话框
在Java代码中实现,点击Button组件时显示带有简单文本信息的气泡对话框。
Component button = findComponentById(ResourceTable.Id_target_component);
button.setClickedListener(new Component.ClickedListener() {
@Override
public void onClick(Component component) {
PopupDialog popupDialog = new PopupDialog(getContext(), component);
popupDialog.setText("This is PopupDialog");
popupDialog.show();
}
});
说明
常用方法的设置需在调用popupDialog.show()之前进行,否则设置无法生效。
图1 点击Button,显示气泡对话框效果图
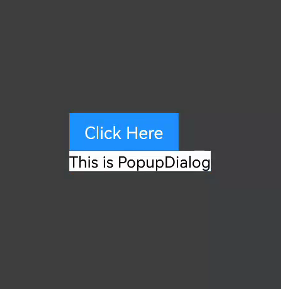
修改对齐模式
popupDialog.setMode(LayoutAlignment.TOP | LayoutAlignment.LEFT);
用户可以使用setMode方法来设置气泡对话框的相对位置。包括左上对齐、左下对齐、右上对齐、右下对齐、上对齐及下对齐。图2 设置左上的对齐效果图
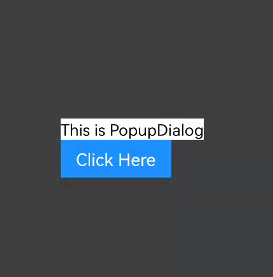
箭头的使用
- 显示箭头:箭头默认不显示。
popupDialog.setHasArrow(true);
图3 显示气泡对话框箭头的效果图
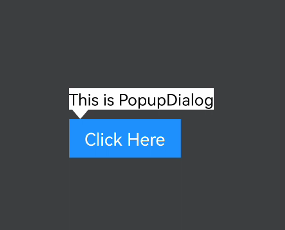
修改箭头属性:可以修改箭头的尺寸和偏移量。
popupDialog.setArrowSize(100, 75); // 设置箭头的宽和高
popupDialog.setArrowOffset(100); // 设置箭头的偏移量
图4 修改气泡对话框箭头的尺寸和偏移量
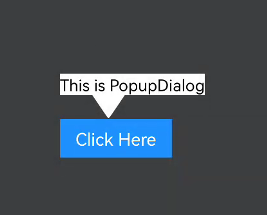
设置气泡对话框的背景色
popupDialog.setBackColor(new Color(0xFFBEEDC7));
图5 设置气泡对话框的背景色
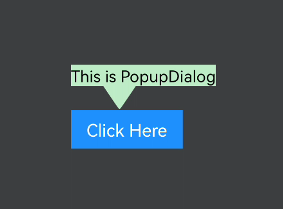
说明
setBackColor不但可以设置内容区域的背景,同时也会修改箭头的背景。
设置相对当前屏幕的显示位置
用户可以使用showOnCertainPosition方法来设置气泡对话框相对当前屏幕的显示位置。
popupDialog.setHasArrow(false);
popupDialog.setMode(LayoutAlignment.LEFT | LayoutAlignment.BOTTOM);// 不生效
popupDialog.showOnCertainPosition(LayoutAlignment.RIGHT, 100, 0);
说明
使用时请注意调用showOnCertainPosition方法会覆盖setMode的设置。
图6 设置气泡对话框相对当前屏幕的显示位置
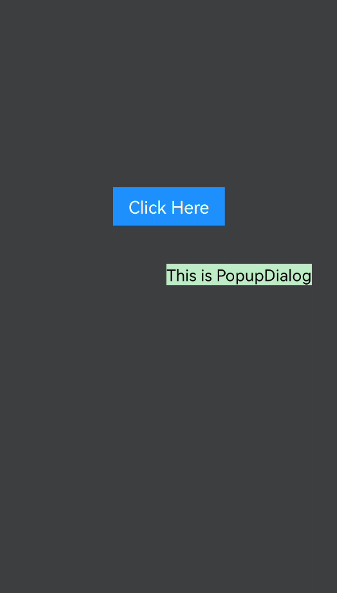
自定义气泡对话框
用户可以使用setCustomComponent来设置气泡对话框所包含的组件。
PopupDialog popupDialog = new PopupDialog(getContext(), component);
RadioContainer radioContainer = new RadioContainer(getContext());
for (int i = 0; i < 3; i++) {
RadioButton radioButton = new RadioButton(getContext());
radioButton.setText("PopupDialog item " + (i + 1));
radioButton.setWidth(460);
radioButton.setHeight(60);
radioButton.setTextSize(40);
ShapeElement bg = new ShapeElement();
bg.setRgbColor(RgbColor.fromArgbInt(Color.getIntColor("#007DFF")));
bg.setCornerRadius(30);
radioButton.setBackground(bg);
radioButton.setTextColorOn(Color.BLACK);
radioButton.setTextColorOff(Color.WHITE);
radioButton.setMarginsLeftAndRight(10, 10);
radioButton.setMarginsTopAndBottom(10, 10);
radioButton.setTextAlignment(TextAlignment.LEFT);
radioContainer.addComponent(radioButton);
}
radioContainer.setPadding(10, 10, 10, 10);
popupDialog.setCustomComponent(radioContainer);
popupDialog.setHasArrow(true);
popupDialog.setArrowOffset(25);
popupDialog.setMode(LayoutAlignment.LEFT | LayoutAlignment.BOTTOM);
popupDialog.setBackColor(new Color(Color.getIntColor("#E8EAEE")));
popupDialog.show();
图7 自定义气泡对话框效果图
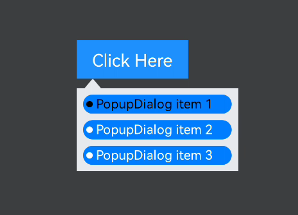